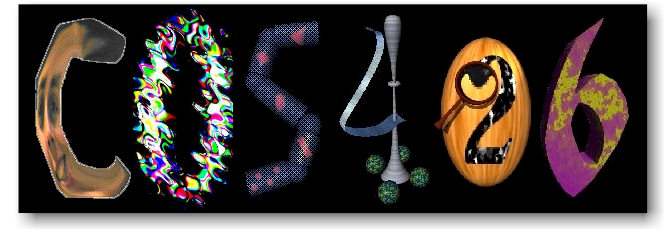
Thanks to Chuck Rose for ideas, code, and data!
Assignment 5: Keyframe Animation
Due: 12/5/99 at 11:59PM
Announcements
12/1 - Be sure to check the course newsgroup regularly in case of announcements..
12/1 - Newer version of the precept slides are available in .ps format
here. (Can someone port them to .pdf?)
11/27 - A bug was found in keyray.c. See /u/cs426/5/BUGS.TXT
for the list of all currently (there's on in there now) known bugs.
Overview
In this assignment you will animate motions for an articulated humanoid
figure. You will be given an articulated 3D model and sets of keyframes
specifying the orientations of articulated joints at specific time steps.
Your job is to interpolate the keyframes smoothly over a time interval
so that the articulated figure performs animated actions (e.g., walks,
dances, etc.).
What You Have to Do
You should implement a program that animates motions of an articulated
figure. The inputs to the program will be:
-
An actor file (.act) specifying an articulated figure as a hierarchy of
limbs connected by rotational joints. You will be given code to read
this file.
The resulting scene graph is a hierarchy of rigid-body links connected
by joints. Each node of the hierarchy represents either a single-parameter
transformation (rotation or translation), an offset (link length), or a
subtree specifying rigid-body geometry (a ray file) in the local coordinate
frame of the joint.
-
A keyframe data file (.key) file specifying the orientation of each joint
at a sequence of time steps. You must write code to read this file
and use the keyframe information to animate the scene graph as a function
of wall-clock time.
Your program should read these files, pop up an OpenGL window, and display
an animated figure moving in real-time. The assignment is worth 15 points.
The following is a list of features that you may implement. The number
in parentheses corresponds to how many points it is worth. Options
in bold are mandatory.
-
(2) Read a keyframe file (.key) and construct a data structure of
your choosing describing joint rotations and translations at keyframes.
-
(1) For each joint, find the corresponding scene graph node whose
tranformation matrix should be controlled by your animation.
-
(2) For each execution of the Idle() function, read the wall-clock
elapsed time (since the beginning of program execution), re-evaluate the
rotation/translation parameters associated with all joints, update the
corresponding scene graph node transformation matrices, and re-draw the
scene.
-
(1) Be able to update joint rotation/translation parameters by linearly
interpolating values specified in the keyframe file.
-
(2) Fit uniform cubic B-splines (with an arbitrary number of control
points) to the keyframe data for each joint using the provided SVD solver,
and be able to update joint parameters by evaluating the B-splines for
each animation time step (rather than linearly interpolating the keyframe
data).
-
(2) Create at least one .actor file referencing a set of .ray files
that describe the non-trivial appearance of your animated character.
-
(2) Create at least one .key file with an interesting motion (e.g.,
an arm wave).
-
(1) Make keyframe animation sequence loop smoothly by fitting a closed
B-spline through the keyframe data. Demonstrate this feature with
a repeating walk cycle.
-
(1) Implement the ability to create new .key files with keyframe data resampled
by your linear/spline interpolation code. Demonstrate this feature
by resampling noisy keyframe data with a fitted B-spline and outputing
a new .key file with smooth motions.
-
(1) Implement the ability to sample the keyframe data at constant time
intervals and dump the frame buffer to a sequence of files. Use movieconvert
on the SGI to create a movie in .mpg file format.
-
(1) Add one or more other kinds of splines (other than uniform cubic B-splines)
and allow the user to switch between them.
-
(1) Add the ability to parse a list of 3-D control points from a file and
generate a camera path spline that the viewpoint flies along.
-
(2) Add the ability to composite two motions specified in separate .key
files onto the same actor at the same time. Augment the .key files
to include a #Start time, and linearly blend between motions for the subsets
of joints in both .key files during transition periods. Demonstrate
this feature with a hand waving motion composited onto a walking cycle.
-
(2) Add the ability to transition between two motions specified in separate
.key files applied to the same actor in successive (slightly overlapping)
time intervals. Augment the .key files to include a #Start time.
Linearly blend between motions for the subsets of joints in both .key files
during overlapping transition periods. Demonstrate this feature with
a transition between two related walk cycles (e.g., a walk and a walk with
a high-five).
-
(2) Add the ability to transition and composite motions in separate .key
files under interactive user control.
-
(2) Augment the .actor files to include hands with fingers containing at
least two joints.
-
(2) Open up another window (or a sub window) and display a visual representation
of the keyframe data, control points, and spline curve for a given degree
of freedom (translation coordinate or rotation angle).
-
(2) Build an interface to step through the key frames, manually, and modify
each DOF, individually, and display the results in realtime. Include
a feature to copy keyframes to ease creation of new .key animations of
arbitrary nature.
-
(3) Augment the above with the capacity to let the user adjust the the
knot spacing of the splines interactively. This implies implementing
non-uniform B-Splines.
-
(?) Impress us with something we hadn't considered...
By implementing all the required features, you get 12 points. There are
many ways to get more points:
-
implementing optional features (as listed above),
-
submitting images for the art contest (1),
-
winning the art contest (1),
-
winning the .ray file contest (1),
-
winning the .key file contest (1),
-
winning the movie file contest (1).
It is possible to get more than 15 points. However, after 15 points, each
point is divided by 2, and after 17 points, each point is divided by 4.
Getting Started
You should use the code available at /u/cs426/5/, on the MECA
machines, as a starting point for your assignment. We provide you with
several files:
-
Makefile.pc, Makefile.sgi - Use the appropriate one for your chosen platform.
-
SVDFit(.cpp)(.h)(.inl) - A high level wrapper for the SVD class. You will
make calls to this to fit splines.
-
MatrixMNTC(.cpp)(.h)(.inl) - A matrix class used with calls to SVDFit.
-
SVD(.cpp)(.h)(.inl) - The low level SVD library class used by SVDFit. You
won't touch this.
-
bmp(.cpp)(.h) - Our familiar BMP tools. Used by the .ray parser to load
textures. You might use this to save output.
-
cone/cube/cylinder/sphere/triangle(.cpp)(.h) - Specific Prim definitions
containining rendering callbacks. You won't touch this.
-
comdef.h - Necessary for SGI's to fake some Windows functionality. You
won't touch this.
-
keyray(.cpp)(.h) - Contains the main GLUT/OpenGL code and your animation/parsing
code. You'll do 99% of your work here.
-
main.cpp - Parses the command line and envokes the main program.
You'll modify this is you add command line options.
-
parser(.cpp)(.h) - A parser for loading .ray files. You'll call to this
to load the .ray files embedded in the .act files.
-
raydata(.cpp)(.h) - All the data type from project #3, with a couple added
structures/features.
-
/data/env.ray - A sample file for use with the -env command line option.
-
/data/muscle.act - A sample actor file.
-
/data/muscle.*.ray - Files describing the muscle actor.
-
/data/stick.act - A sample actor file.
-
/data/stick.*.ray - Files describing the stick actor.
-
/data/*.key - Sample keyframe files.
The only file you will have to touch is keyray.c. There you will
find various functions that you have to implement. See the comments in
the code for details.
After you copy the provided files to your directory, the first thing
to do is compile the program.
-
If you are developing on a PC, running Microsoft Visual C++, procees as
follows:
% copy Makefile.pc Makefile
% "c:\Program Files\Microsoft Visual Studio\VC98\Bin\vcvars32"
% nmake
If you receive errors about missing glut libraries, download glutdlls.zip.
-
If you are developing on an SGI machine:
% cp Makefile.sgi Makefile
% make depend
% make
An executable called keyray or keyray.exe will be compiled
and linked. Run this with -help on the command line to see a usage description.
What to Submit
You should submit:
-
the complete source code with a Makefile that works on the MECA
machines,
-
any interesting .act (and associated .ray) files you created.
-
any .key files you created.
-
any movies for the art contest
-
the images for the art contest, and
-
a writeup.
The writeup should be a HTML document called assignment5.html which
may include other documents or pictures. It should be brief, describing
what you have implemented, what works and what doesn't, how you created
your .act and .key files, how you created the art contest images, and any
relevant instructions on how to compile and run your program.
Make sure the source code compiles in the MECA
workstations. If it doesn't, you will have to attend to a grading session
with a TA, and your grade will suffer.
Art Contest
Be sure to label all art contest submission files with the prefix "art_"
(e.g., art_foo.key, art_foo.act).
Always remember the late
policy and the collaboration
policy.
Last update: 11/10/99
10:17 AM